In this post we’ll explore how static typing can effect the performance of your projects. We will also try to figure out if implementing this into your codebase is worth the effort, or negligible at best.
GDScript, Godot’s built-in scripting language, is gradually typed.
This means that GDScript offers the best of both type systems: the flexibility of dynamic typing and when you choose to use it, the performance benefits of static typing.
# Example of a dynamically typed variable
var player_name = "N. Brazil"
# Converting player_name to an integer
player_name = 42
# Example of a statically typed variable
var player_input: string = "Inspect the dagger"
# This line will throw error
player_input = 1996
# Alternate method to declare statically typed variables
# These will all be typed to Vector3
var a: Vector3 = Vector3.ZERO
var b := a
var c := Vector3.ZERO
Thank you to @nate.moore.codes for providing alternate method for declaration
This allows us to prototype code quickly without the worry of variable declarations or overwriting variables with different types.
Then during an optimisation phase you’ll be able to add static type declarations in performance-critical systems (or everywhere!).
Performance Benchmark
To demonstrate the impact of static typing, let’s look at some real-world performance comparisons. We’ll test three common operations: addition, multiplication, and vector calculations.
Here is a bit of the code used for the benchmark, the full source is available on GitHub.
# Iteration counts
const ITERATIONS: int = 25_000_000
const WARMUP_ITERATIONS: int = 10_000
# Test variables
var untyped_int = 0
var typed_int: int = 0
var untyped_float = 0.0
var typed_float: float = 0.0
var untyped_vector = Vector2.ZERO
var typed_vector: Vector2 = Vector2.ZERO
var untyped_string = ""
var typed_string: String = ""
Benchmark Results
In-Editor
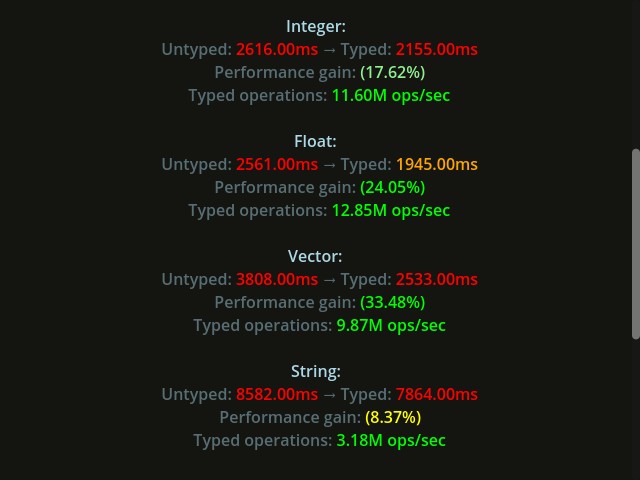
Debug Build
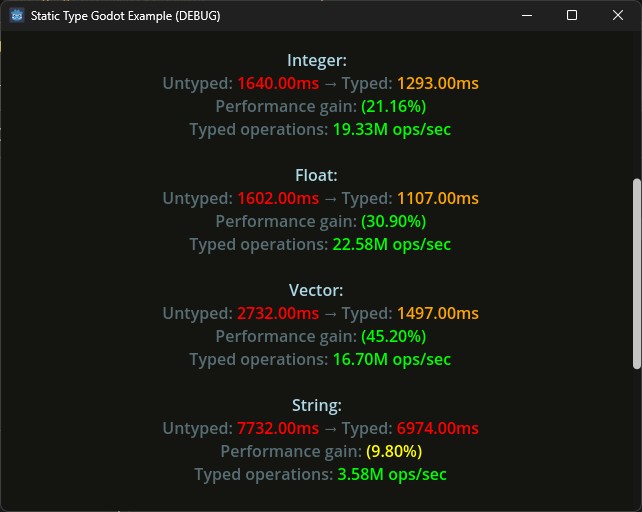
Release Build
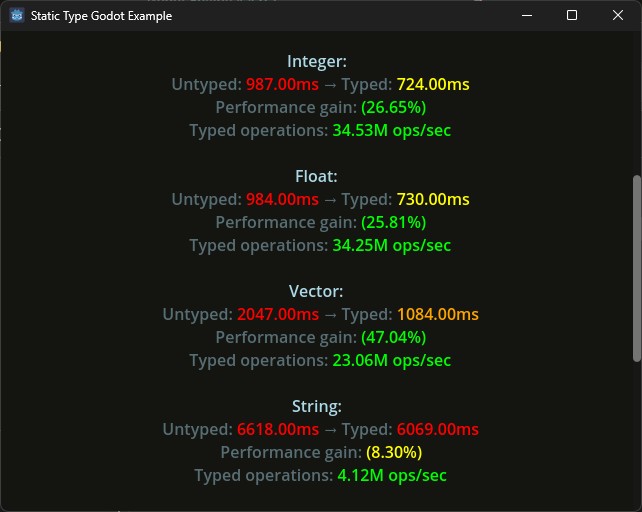
The performance gains are particularly impressive with Vector2 operations, showing up to a 47.04% improvement in release builds!
In-Editor Type Annotations
Implementing static typing in your Godot project is straightforward.
Step 1: Enable “Add Type Hints” in the editor settings
- Navigate to the menu bar at the top of your Godot instance
- Click Editor > Editor Settings
- Search for “type_hints”
- Enable “text_editor/completion/add_type_hints”
Now, the editor automatically pre-fill known annotations as you type your code.
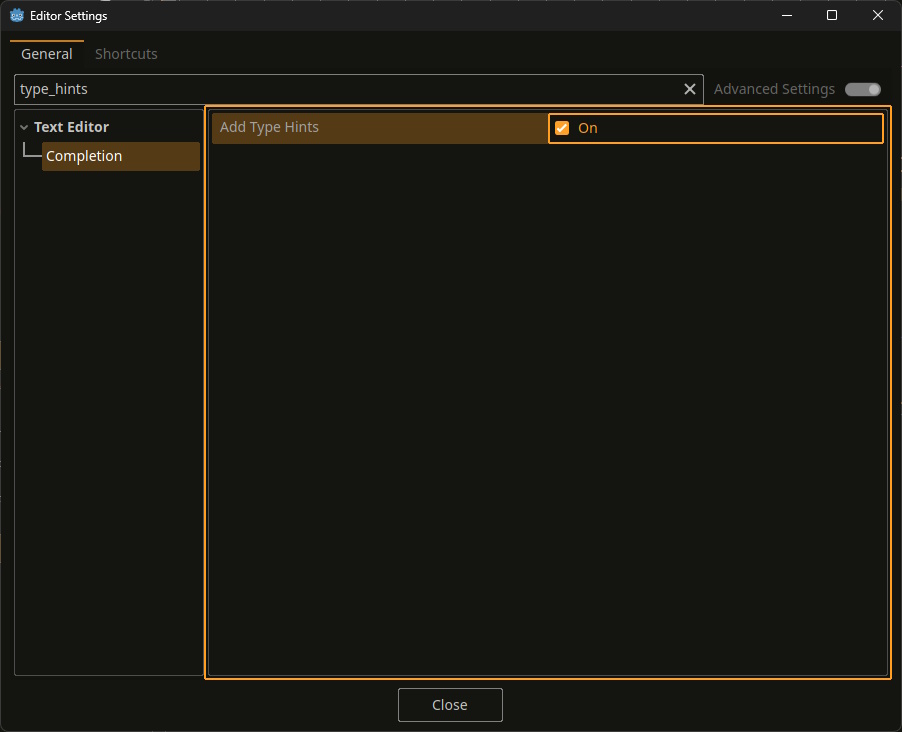
Step 2: Add type annotations to your variables
var player_health: int = 100
var player_position: Vector2 = Vector2.ZERO
var is_jumping: bool = false
Step 3: Use type annotations in function definitions
# Calculate the total amount of damage
func total_damage(base_damage: float, multiplier: float) -> float:
return base_damage * multiplier
Step 4: Look for red error indicators in the editor
These indicate type safety violations that Godot can detect at parse time. You’ll see specific error messages like “Cannot pass a value of type String
as int
” when type mismatches occur.
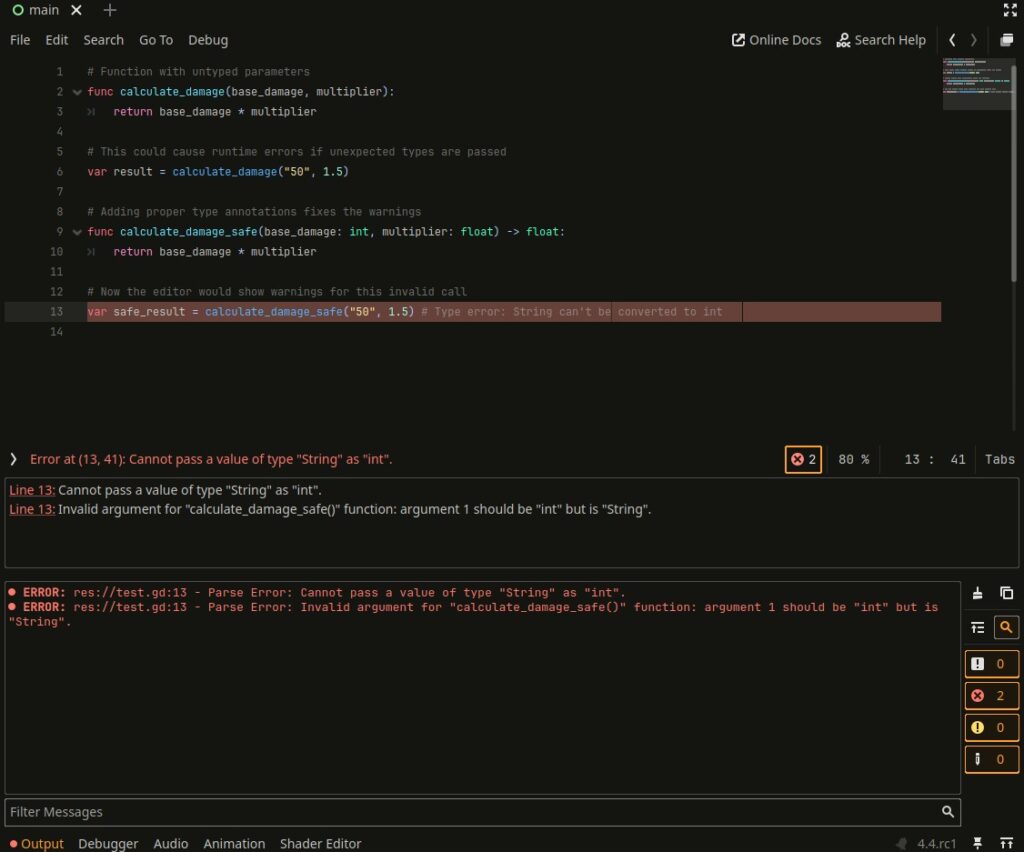
Adding proper type annotations not only prevents these errors but also improves code quality and helps catch bugs before runtime.
So is it worth the effort to implement static typing into your project? Up to you, but I think so!
Leave a Reply